SqlCommandBuilder
Setting up the SQL Connection
-
Start by adding "System.Data" to your references. After that add "System.Data.SqlClient" to your using statements.
-
Create a new SqlConnection object and provide it with a connection string. Substitute your own parameters within the brackets.
i.e: SqlConnection newCon = new SqlConnection("Server=[Ip Address];Database=[Database Name];User Id=[DB Username];Password=[DB Password];");
-
Next call the open function for your connection.
i.e: newCon.Open();
- You should now have a connection to your database.
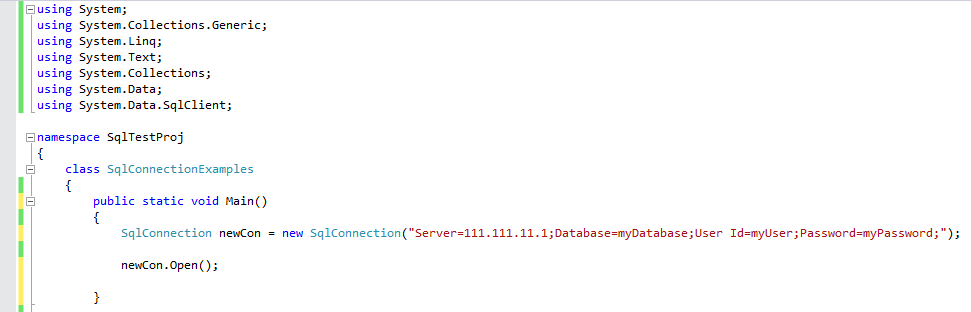
Adding to the Database
-
Begin by creating a SqlCommand object and setting the connection to the one you made above.
i.e: SqlCommand myCommand = new SqlCommand();
myCommand.connection = newCon;
-
Next set the command text for the add, and the parameters. Substitute your own parameters within the brackets.
i.e: myCommand.CommandText = "INSERT INTO [Table Name] ([Column Name]) values (@[Column Name])";
myCommand.Parameters.Add("@[Column Name]", [Column Type]).Value = "Value";
-
Execute the command.
i.e: myCommand.ExecuteNonQuery();
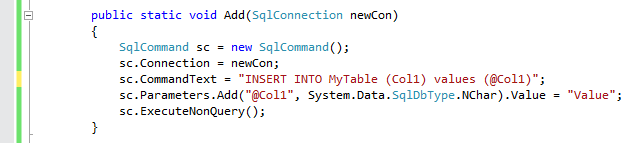
Reading from the Database
-
Begin by creating a SqlCommand object and setting the connection to the one you made above.
i.e: SqlCommand myCommand = new SqlCommand();
myCommand.connection = newCon;
-
Next set the command text for the read. Substitute your own parameters within the brackets.
i.e: myCommand.CommandText = "SELECT * FROM [Table Name]";
-
For this example we will be using a DataReader to read the lines from the table.
Create a SqlDataReader object and set it equal to the execution statement of your command.
i.e: SqlDataReader sdr = myCommand.ExecuteReader();
-
Set up a while loop to run while the SqlDataReader reads from the table, and fetch the values from specified columns. Substitute your own parameters within the brackets.
i.e: while (sdr.Read())
{
Console.Write(sdr["[Column Name]"].ToString().Trim());
}
-
Close the SqlDataRader.
i.e: sdr.Close();
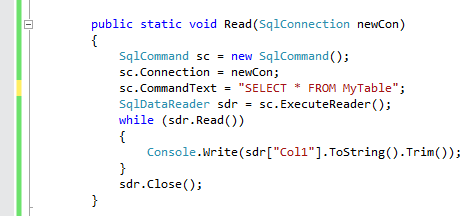
Deleting from the Database
-
Begin by creating a SqlCommand object and setting the connection to the one you made above.
i.e: SqlCommand myCommand = new SqlCommand();
myCommand.connection = newCon;
-
Next set the command text for the delete. Substitute your own parameters within the brackets.
i.e: myCommand.CommandText = "DELETE FROM [Table Name] WHERE [Column Name]='Value'";
-
Execute the command. If you would like, you can capture the number of rows deleted.
i.e: int rowsAffected = myCommand.ExecuteNonQuery();
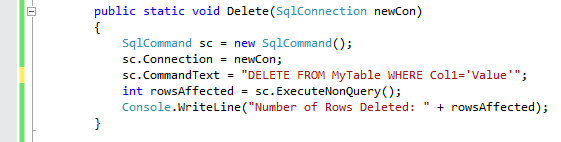
Setting up a SqlCommandBuilder for the Database
-
Begin by creating a SqlDataAdapter object, a DataTable object and a SqlCommandBuilder Object
i.e: SqlDataAdapter da = null;
DataTable dtOne = new DataTable();
SqlCommandBuilder scb = null;
-
Next, create a SqlCommand object and set the connection to the one you made above.
i.e: SqlCommand myCommand = new SqlCommand();
myCommand.connection = newCon;
-
Set the command text as if you are doing a read. Substitute your own parameters within the brackets.
i.e: myCommand.CommandText = "SELECT * FROM [Table Name]";
-
Set the SqlDataAdapter to a new SqlDataAdapter object and pass in your SqlCommand.
i.e: da = new SqlDataAdapter(sc);
-
Next, set the SqlCommandBuilder to a new SqlCommandBuilder object and pass in the SqlDataAdapter.
i.e: scb = new SqlCommandBuilder(da);
This will automatically set up the rest of the commands for working the the table.
-
Use the SqlDataAdapter to fill your DataTable.
i.e: da.Fill(dtOne);
-
You may now edit the data within your DataTable. Adding, Modifying and Deleting will all work.
When you wish to submit your changes simply call the SqlDataAdapters update command and pass in the DataTable.
i.e: da.Update(dtOne);
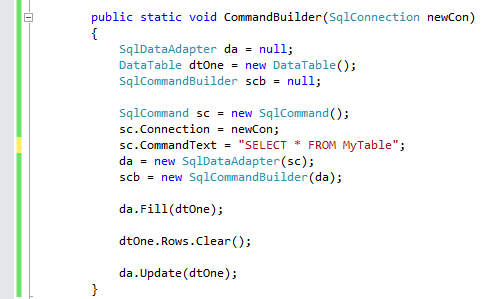